WiFi Web Server LED Blink
A simple web server that lets you blink an LED via the web.
This sketch will create a new access point (with no password).
It will then launch a new server and print out the IP address
to the Serial monitor. From there, you can open that address in a web browser
to turn on and off the LED on pin 13.
If the IP address of your shield is yourAddress:
http://yourAddress/H turns the LED on
http://yourAddress/L turns it off
created 25 Nov 2012
by Tom Igoe
adapted to WiFi AP by Adafruit
*/
#include
#include
#include 'arduino_secrets.h'
///////please enter your sensitive data in the Secret tab/arduino_secrets.h
char ssid[]= SECRET_SSID;// your network SSID (name)
char pass[]= SECRET_PASS;// your network password (use for WPA, or use as key for WEP)
int keyIndex =0;// your network key Index number (needed only for WEP)
int led = LED_BUILTIN;
int status = WL_IDLE_STATUS;
WiFiServer server(80);
voidsetup(){
//Initialize serial and wait for port to open:
Serial.begin(9600);
while(!Serial){
;// wait for serial port to connect. Needed for native USB port only
}
Serial.println('Access Point Web Server');
pinMode(led,OUTPUT);// set the LED pin mode
// check for the presence of the shield:
if(WiFi.status() WL_NO_SHIELD){
Serial.println('WiFi 101 Shield not present');
// don't continue
while(true);
}
// by default the local IP address of will be 192.168.1.1
// you can override it with the following:
// WiFi.config(IPAddress(10, 0, 0, 1));
// print the network name (SSID);
Serial.print('Creating access point named: ');
Serial.println(ssid);
// Create open network. Change this line if you want to create a WEP network:
status = WiFi.beginAP(ssid);
if(status != WL_AP_LISTENING){
Serial.println('Creating access point failed');
// don't continue
while(true);
}
// wait 10 seconds for connection:
delay(10000);
// start the web server on port 80
server.begin();
// you're connected now, so print out the status
printWiFiStatus();
}
voidloop(){
// compare the previous status to the current status
if(status != WiFi.status()){
// it has changed, so update the variable
status = WiFi.status();
if(status WL_AP_CONNECTED){
byte remoteMac[6];
// a device has connected to the AP
Serial.print('Device connected to AP, MAC address: ');
WiFi.APClientMacAddress(remoteMac);
printMacAddress(remoteMac);
}else{
// a device has disconnected from the AP, and we are back in listening mode
Serial.println('Device disconnected from AP');
}
}
WiFiClient client = server.available();// listen for incoming clients
if(client){// if you get a client,
Serial.println('new client');// print a message out the serial port
String currentLine =';// make a String to hold incoming data from the client
while(client.connected()){// loop while the client's connected
if(client.available()){// if there are bytes to read from the client,
char c = client.read();// read a byte, then
Serial.write(c);// print it out the serial monitor
if(c 'n'){// if the byte is a newline character
// if the current line is blank, you got two newline characters in a row.
// that's the end of the client HTTP request, so send a response:
if(currentLine.length()0){
// HTTP headers always start with a response code (e.g. HTTP/1.1 200 OK)
// and a content-type so the client knows what's coming, then a blank line:
client.println('HTTP/1.1 200 OK');
client.println('Content-type:text/html');
client.println();
// the content of the HTTP response follows the header:
client.print('Click '/H'>here turn the LED on
');
client.print('Click '/L'>here turn the LED off
');
// The HTTP response ends with another blank line:
client.println();
// break out of the while loop:
break;
}
else{// if you got a newline, then clear currentLine:
currentLine =';
}
}
elseif(c !='r'){// if you got anything else but a carriage return character,
currentLine += c;// add it to the end of the currentLine
}
// Check to see if the client request was 'GET /H' or 'GET /L':
if(currentLine.endsWith('GET /H')){
digitalWrite(led,HIGH);// GET /H turns the LED on
}
if(currentLine.endsWith('GET /L')){
digitalWrite(led,LOW);// GET /L turns the LED off
}
}
}
// close the connection:
client.stop();
Serial.println('client disconnected');
}
}
void printWiFiStatus(){
// print the SSID of the network you're attached to:
Serial.print('SSID: ');
Serial.println(WiFi.SSID());
// print your WiFi 101 Shield's IP address:
IPAddress ip = WiFi.localIP();
Serial.print('IP Address: ');
Serial.println(ip);
// print the received signal strength:
long rssi = WiFi.RSSI();
Serial.print('signal strength (RSSI):');
Serial.print(rssi);
Serial.println(' dBm');
// print where to go in a browser:
Serial.print('To see this page in action, open a browser to http://');
Serial.println(ip);
}
void printMacAddress(byte mac[]){
for(int i =5; i >=0; i--){
if(mac[i]<16){
Serial.print('0');
}
Serial.print(mac[i],HEX);
if(i >0){
Serial.print(':');
}
}
Serial.println();
}
Located in Southern California, this Harrah's resort has one of the biggest and most active casinos in the region, with over 1,700 slot machines and nearly 60 exciting table games, including poker and blackjack. Harrah's does charge $11.95 (as of Feb) for 24 hour internet access. The Venetian is an app. 5-10 minute walk from Harrah's (if indeed there is free wifi in the food court.I don't know). For us, I feel it is worth it to pay the daily fee, rather than dragging the laptop/netbook around.
Android 10 introduces support for the Wi-Fi Alliance's(WFA) Wi-Fi Protected Access version 3 (WPA3) and Wi-Fi Enhanced Openstandards. For moreinformation, seeSecurity on the WFA site.
WPA3 is a new WFA security standard for personal and enterprisenetworks. It aims to improve overall Wi-Fi security by using modern securityalgorithms and stronger cipher suites. WPA3 has two parts:
- WPA3-Personal: Uses simultaneous authentication of equals (SAE)instead of pre-shared key (PSK), providing users with stronger securityprotections against attacks such as offline dictionary attacks, keyrecovery, and message forging.
- WPA3-Enterprise: Offers stronger authentication and link-layerencryption methods, and an optional 192-bit security mode for sensitivesecurity environments.
Wi-Fi Enhanced Open is a new WFA security standard for publicnetworks based on opportunistic wireless encryption (OWE). It providesencryption and privacy on open, non-password-protected networks in areas such ascafes, hotels, restaurants, and libraries. Enhanced Open doesn't provideauthentication.
WPA3 and Wi-Fi Enhanced Open improve overall Wi-Fi security, providing betterprivacy and robustness against known attacks. As many devices don't yet supportthese standards or haven't yet had software upgrades to support these features,WFA has proposed the following transition modes:
- WPA2/WPA3 transition mode: The serving access point supports bothstandards concurrently. In this mode, Android 10devices use WPA3 to connect, and devices running Android 9 or lower useWPA2 to connect to the same access point.
- OWE transition mode: The serving access point supports both OWE and openstandards concurrently. In this mode, Android 10devices use OWE to connect, and devices running Android 9 or lower connectto the same access point without any encryption.
https://softlearn.mystrikingly.com/blog/dolphins-pearl-online. WPA3 and Wi-Fi Enhanced Open are supported only in client mode.
Harrahs Wifi Access Code
Implementation
To support WPA3 and Wi-Fi Enhanced Open, implement the supplicant HAL interfacedesign language (HIDL) provided in the Android Open Source Project (AOSP) athardware/interfaces/wifi/supplicant/1.3/
.
The following are required to support WPA3 and OWE: Casinos in south florida.
Linux kernel patches to support SAE and OWE
- cfg80211
- nl80211
wpa_supplicant
with support for SAE, SUITEB192 and OWEWi-Fi driver with support for SAE, SUITEB192, and OWE
Wi-Fi firmware with support for SAE, SUITEB192, and OWE
Wi-Fi chip with support for WPA3 and OWE
Public API methods are available in Android 10 to allowapps to determine device support for these features:
WifiConfiguration.java
contains new key management types, as well as pairwise ciphers, group ciphers,group management ciphers, and Suite B ciphers, which are required for OWE,WPA3-Personal, and WPA3-Enterprise.
Enabling WPA3 and Wi-Fi Enhanced Open
To enable WPA3-Personal, WPA3-Enterprise, and Wi-Fi Enhanced Open in the Androidframework:
Quick hits online slots. Ruby fortune contact. WPA3-Personal: Include the
CONFIG_SAE
compilation option in thewpa_supplicant
configuration file.WPA3-Enterprise: Include the
CONFIG_SUITEB192
andCONFIG_SUITEB
compilation options in thewpa_supplicant
configuration file.Wi-Fi Enhanced Open: Include the
CONFIG_OWE
compilation option in thewpa_supplicant
configuration file.
If WPA3-Personal, WPA3-Enterprise, or Wi-Fi Enhanced Open aren't enabled, userswon't be able to manually add, scan, or connect to these types of networks.
Validation
Platinum play casino app. To test your implementation, run the following tests.
Unit tests
Harrahs Wifi Access Codes
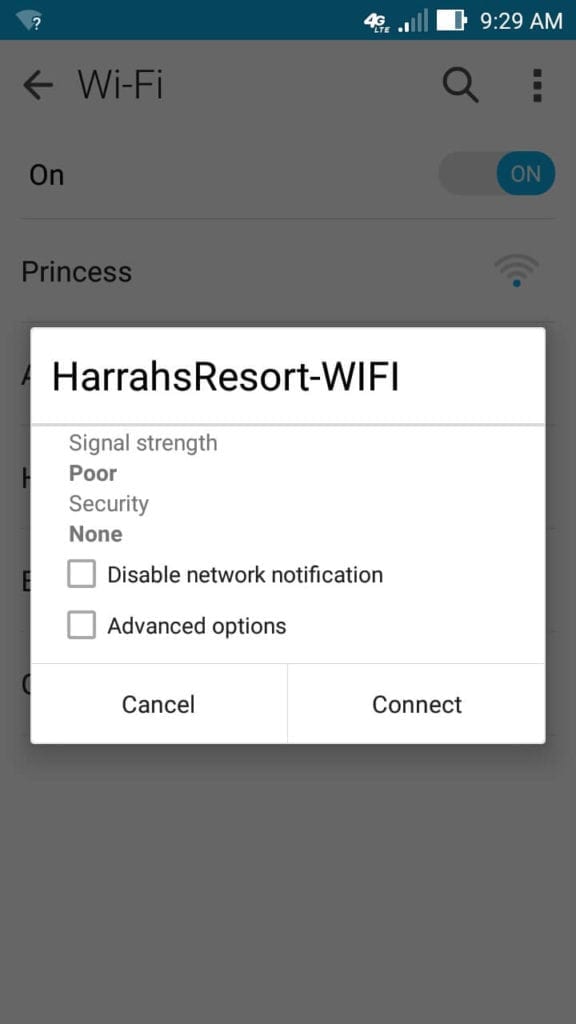
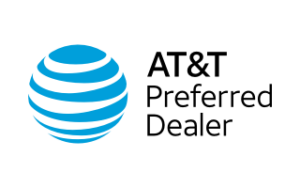
RunSupplicantStaIfaceHalTest
to verify the behavior of the capability flags for WPA3 and OWE.
RunWifiManagerTest
to verify the behavior of the public APIs for this feature.
Integration test (ACTS)
To run an integration test, use the Android Comms Test Suite (ACTS) file,WifiWpa3OweTest.py
,located in tools/test/connectivity/acts/tests/google/wifi
.
VTS tests
RunVtsHalWifiSupplicantV1_3Host
to test the behavior of the supplicant HAL 1.3.